Understanding Callback Functions in Python
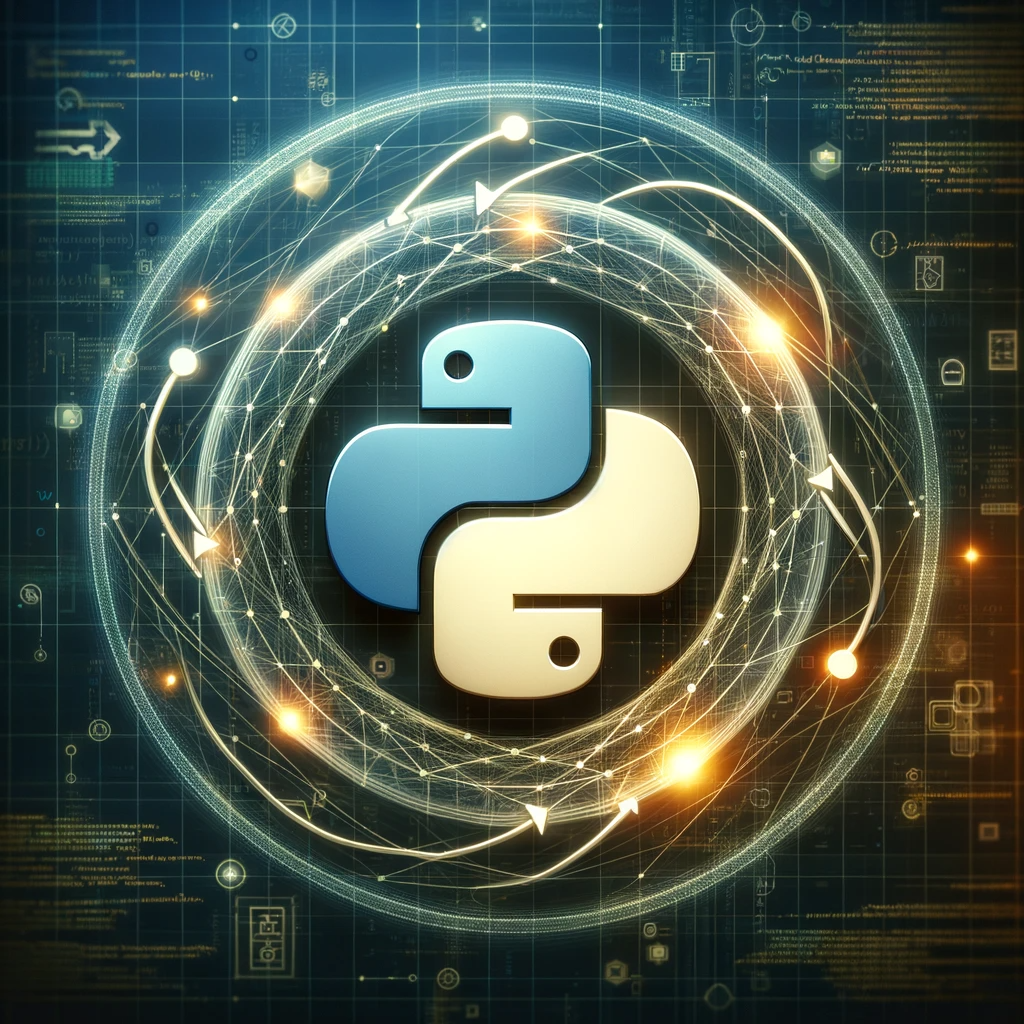
Understanding Callback Functions in Python
Callback functions are a crucial concept in Python, especially useful in asynchronous programming, event handling, and function customization. Let’s delve into their nature and application.
What is a Callback Function?
- Callback Function: A function passed into another as an argument, executed at a specific point within the host function. It’s a code piece for later execution, not immediate.
Key Use Cases
- Asynchronous Operations: For signaling task completion, like post-data-fetch operations.
- Event Listeners: In GUI or web development, they respond to user interactions like clicks or key presses.
- Higher-Order Functions: Used in functions like
map()
,filter()
, andsorted()
to define operation behavior.
Python Example: Simple Callback
Consider process_data
, which processes data and uses a callback:
def process_data(data, callback):
# Example processing
processed_data = data * 2
callback(processed_data) # Execute the callback
def print_result(result):
print(f"Processed result: {result}")
# Using the callback
process_data(5, print_result)
Here, print_result is the callback, executed post-processing by process_data.
Best Practices for Callbacks
- Clear Naming: For indicating purpose.
- Robust Error Handling: To manage different execution contexts.
- Simplicity: Avoiding convoluted logic for better readability.
- Documentation: Detailing expected signature and behavior.
Implementing Error Handling
Robust error management in callbacks is vital. Here’s an enhanced print_result:
def process_data(data, callback):
try:
processed_data = data * 2
callback(processed_data)
except Exception as e:
print(f"An error occurred: {e}")
def print_result(result):
try:
if result < 0:
raise ValueError("Result is negative")
print(f"Processed result: {result}")
except ValueError as ve:
print(f"Error in callback: {ve}")
process_data(5, print_result)
process_data(-3, print_result) # Triggers error handling
Simplifying Callback Logic
Complex callback logic can be a maintenance hurdle.
Before Simplification
def complex_callback(data):
# Multiple steps that make the callback complex
step1 = data + 10
step2 = step1 / 2
if step2 > 20:
step3 = step2 * 3
else:
step3 = step2 + 5
print(f"Final result: {step3}")
After Simplification
Breaking down into smaller functions:
def step_one(data):
return data + 10
def step_two(data):
return data / 2
def step_three(data):
return data * 3 if data > 20 else data + 5
def simple_callback(data):
result1 = step_one(data)
result2 = step_two(result1)
final_result = step_three(result2)
print(f"Final result: {final_result}")
Now, simple_callback is more readable and manageable.
Conclusion
Callbacks in Python are powerful for flexibility and reusability but require careful use to avoid complexity. They are a fundamental part of asynchronous programming and event-driven systems, offering a robust way to customize function behavior. By following best practices, you can use them effectively.